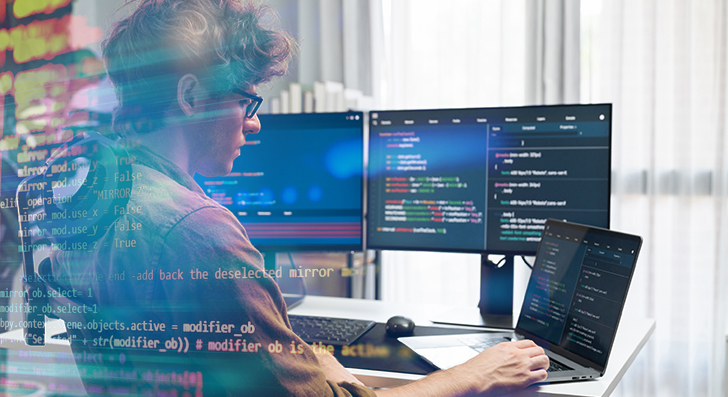
Scalability usually means your application can deal with advancement—additional end users, more details, plus more website traffic—with no breaking. Like a developer, building with scalability in your mind saves time and worry afterwards. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability is just not a little something you bolt on later on—it ought to be element of your prepare from the beginning. A lot of applications fall short when they grow rapidly because the initial design can’t take care of the additional load. Like a developer, you might want to Believe early about how your technique will behave stressed.
Begin by coming up with your architecture to become versatile. Stay clear of monolithic codebases in which anything is tightly connected. In its place, use modular style or microservices. These designs crack your application into smaller sized, impartial elements. Each individual module or services can scale on its own without impacting The full procedure.
Also, consider your database from day just one. Will it have to have to handle 1,000,000 buyers or just a hundred? Choose the proper form—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even when you don’t need them however.
Yet another critical place is to stay away from hardcoding assumptions. Don’t generate code that only works under present problems. Contemplate what would materialize In the event your person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use design and style patterns that aid scaling, like information queues or party-pushed programs. These support your app manage a lot more requests with no receiving overloaded.
Once you Establish with scalability in your mind, you're not just getting ready for success—you're reducing long term headaches. A well-prepared system is less complicated to take care of, adapt, and increase. It’s far better to prepare early than to rebuild afterwards.
Use the appropriate Database
Choosing the right database is usually a critical Portion of developing scalable applications. Not all databases are designed the identical, and using the Completely wrong one can slow you down or perhaps induce failures as your app grows.
Begin by being familiar with your knowledge. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is an effective in good shape. These are typically robust with interactions, transactions, and consistency. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle additional website traffic and info.
In the event your info is a lot more flexible—like consumer activity logs, merchandise catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling significant volumes of unstructured or semi-structured information and might scale horizontally more effortlessly.
Also, look at your study and publish styles. Have you been performing numerous reads with much less writes? Use caching and read replicas. Do you think you're managing a hefty publish load? Take a look at databases that may take care of superior publish throughput, or simply event-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also sensible to Assume in advance. You might not need State-of-the-art scaling options now, but choosing a database that supports them indicates you gained’t want to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your data dependant upon your entry designs. And generally watch databases functionality while you increase.
Briefly, the appropriate databases will depend on your application’s composition, velocity requires, And exactly how you be expecting it to improve. Acquire time to choose correctly—it’ll preserve plenty of problems later on.
Enhance Code and Queries
Quickly code is key to scalability. As your app grows, each individual compact hold off adds up. Badly written code or unoptimized queries can decelerate general performance and overload your system. That’s why it’s important to Establish successful logic from the beginning.
Commence by writing clean up, uncomplicated code. Keep away from repeating logic and remove anything avoidable. Don’t select the most advanced Remedy if a simple a person performs. Keep your features quick, focused, and simple to test. Use profiling applications to seek out bottlenecks—spots exactly where your code takes way too lengthy to operate or makes use of too much memory.
Upcoming, examine your databases queries. These usually gradual items down over the code alone. Ensure each query only asks for the info you really have to have. Stay away from Find *, which fetches every little thing, and instead decide on unique fields. Use indexes to speed up lookups. And avoid undertaking a lot of joins, Specifically throughout large tables.
Should you see exactly the same facts being requested time and again, use caching. Store the final results temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your application extra efficient.
Remember to examination with massive datasets. Code and queries that do the job fine with 100 information may possibly crash if they have to take care of one million.
In short, scalable apps are rapidly applications. Maintain your code limited, your queries lean, and use caching when wanted. These ways help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle far more end users plus much more website traffic. If all the things goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s where by load balancing and caching are available. Both of these equipment aid keep the application quickly, stable, and scalable.
Load balancing spreads incoming visitors across various servers. In place of one particular server undertaking each of the perform, the load balancer routes customers to different servers based on availability. This suggests no one server will get overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info temporarily so it could be reused swiftly. When customers ask for a similar facts once more—like an item website page or perhaps a profile—you don’t need to fetch it with the database when. It is possible to serve it with the cache.
There are two popular forms of caching:
1. Server-facet caching (like Redis or Memcached) merchants data in memory for rapid access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents close to the consumer.
Caching reduces database load, increases speed, and would make your application more productive.
Use caching for things which don’t modify normally. And often ensure that your cache is updated when knowledge does improve.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they help your application take care of more consumers, keep fast, and Recuperate from challenges. If you propose to develop, you require both.
Use Cloud and Container Resources
To create scalable purposes, you need resources that allow your application develop simply. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and expert services as you would like them. You don’t have to purchase hardware or guess long run ability. When targeted visitors improves, you can add much more sources with just a few clicks or immediately making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms click here also supply providers like managed databases, storage, load balancing, and safety resources. It is possible to target constructing your app as opposed to handling infrastructure.
Containers are An additional essential Device. A container deals your app and everything it really should operate—code, libraries, options—into 1 unit. This can make it effortless to move your application in between environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app uses many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part within your app crashes, it restarts it automatically.
Containers also help it become straightforward to independent parts of your application into solutions. You could update or scale areas independently, that is perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container instruments indicates you may scale quickly, deploy conveniently, and Recuperate immediately when difficulties materialize. If you prefer your app to improve with out boundaries, start employing these applications early. They conserve time, lower danger, and make it easier to stay focused on making, not correcting.
Check Anything
In the event you don’t keep an eye on your software, you received’t know when issues go Completely wrong. Monitoring will help the thing is how your application is carrying out, place difficulties early, and make greater conclusions as your application grows. It’s a important Portion of making scalable systems.
Begin by tracking standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and providers are undertaking. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this knowledge.
Don’t just watch your servers—watch your application much too. Regulate how much time it takes for customers to load pages, how often mistakes occur, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s occurring inside your code.
Create alerts for crucial difficulties. As an example, If the reaction time goes earlier mentioned a limit or even a support goes down, you ought to get notified right away. This assists you repair problems fast, normally right before people even detect.
Monitoring can also be useful after you make variations. When you deploy a whole new characteristic and see a spike in glitches or slowdowns, it is possible to roll it again ahead of it leads to real problems.
As your app grows, traffic and details enhance. Without having checking, you’ll overlook signs of issues right up until it’s as well late. But with the ideal equipment in place, you keep in control.
Briefly, monitoring allows you maintain your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about knowing your system and making certain it works properly, even stressed.
Ultimate Views
Scalability isn’t just for significant companies. Even modest apps need to have a solid foundation. By coming up with cautiously, optimizing correctly, and using the proper applications, you'll be able to Establish apps that increase effortlessly without having breaking stressed. Start tiny, Imagine massive, and Establish wise.